Topic 1: Computational thinking
About
Students are expected to develop a set of computational thinking skills that enable them to design, implement and analyse algorithms for solving problems. Students are expected to be familiar with and use the Programming Language Subset (PLS) document provided on the GCSE in Computer Science section of our website. The flowchart symbols students are expected to be familiar with and use are the basic flowchart symbols.
So...on this page there are the content for this topic. Click the links below for help on it
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
Decomposition & Abstraction
PART 1- Decomposition involves analysing a complex problem or system and breaking it down into smaller parts that are more manageable and easy to understand. The smaller parts can then be examined and solved, or designed individually, as they are simpler to work with.
If a problem is not decomposed, it is much harder to solve. Dealing with a complex problem is much more difficult than breaking a problem down into subproblems and solving them, one at a time. Smaller problems are easier to understand and can be examined in more detail.
For example, suppose that a crime has been committed. Solving a crime can be a very complex problem as there are many things to consider.
A police officer would need to know the answer to a series of smaller problems:
-
what crime was committed
-
when the crime was committed
-
where the crime was committed
-
what evidence there is
-
if there were any witnesses
-
if there have recently been any similar crimes
The complex problem of the committed crime has now been broken down into simpler problems that can be examined individually, in detail. Once the individual information has been gathered and collated, the police officer may be able to solve the crime.
PART 1 ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
PART 2- Abstraction is the process of filtering out - essentially ignoring - the characteristics of problems that are not needed in order to concentrate on those that are needed. It is also the filtering out of specific details. From this, an idea of what is to be solved can be created.
Abstraction allows programmers to create a general idea of what the problem is and how to solve it. The process instructs them to remove all specific detail and any patterns that will not help find a solution. An idea of the problem can then be formed. This idea is known as a ‘model’.
Consider the problem of how a program might be required to calculate the area of any rectangle. All rectangles share general characteristics:
-
a width
-
a height
-
a formula to calculate area: area = width × height
When abstracting, certain details are discarded but others are kept:
-
all rectangles have a width, but for the program design the actual rectangle width is not needed
-
all rectangles have a height, but for the program design the actual rectangle height is not needed
-
area is always width × height
-
To solve this problem, the program needs to be able to input a width and a height, then calculate the area from those numbers. The actual numbers are irrelevant - they change with every rectangle - and so are discarded.
An example of abstraction is the London Underground map. It details tube and rail lines and the stations that are on them. That is all that is required for passengers to be able to plan a journey from one station to another. Other details, such as real geographical location, distance between stations, depth underground and number of platforms are not included. They are not relevant to journey planning on the Underground.
PART 2 ACTIVITY >> WATCH THIS VIDEO or THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 3- Algorithms
An algorithm is a logical, step-by-step process for solving a problem. Algorithms are normally written using one of the following conventions:
-
pseudo-code
-
flowcharts
-
written descriptions
-
program code
An algorithm should be seen as a starting point before writing a program. It should include the required programming constructs to solve the problem, ie sequence, selection and iteration. The finished program should follow the steps the algorithm describes.
Before an algorithm can be designed, it is important to check that the problem is completely decomposed.
The decomposed problem should consider the following questions:
-
What are the inputs into the problem?
-
What will be the outputs of the problem?
-
In what order do instructions need to be carried out?
-
What decisions need to be made to solve the problem?
-
Are any areas of the problem repeated?
-
Only when a problem is properly decomposed and understood can an algorithm design begin.
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 4- Flowcharts
A flowchart is a diagram that shows an overview of a program. Flowcharts normally use standard symbols to represent the different types of instructions. These symbols are used to construct the flowchart and show the step-by-step solution to the problem. Flowcharts are sometimes known as flow diagrams.
Common flowchart symbols
Flowcharts can be used to plan out programs. This simple flowchart maps out an algorithm for a program that prints the numbers 1 to 10:
Advantages and disadvantages of using flowcharts
Designing an algorithm using a flowchart has advantages because:
-
it is easy to see how a program flows
-
flowcharts follow an international standard - it is easy for any flowchart user to pick up a diagram and understand it
Flowcharts also have their disadvantages:
-
with a large program, the diagrams can become huge and therefore difficult to follow
-
any changes to the design may mean a lot of the diagram has to be redrawn
PART 4- ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 5- Pseudocode
Most programs are developed using programming languages. These languages have specific syntax that must be used so that the program will run properly.
Pseudo-code is not a programming language. Instead, it is a simple way of describing a set of programming instructions in a manner that resembles a programming language. Pseudo-code has its own syntax, some of which is very similar to many actual programming languages. Any algorithms designed using pseudo-code will not run unless they are converted into an actual programming language.
This simple pseudo-code algorithm asks a user to input what their favourite subject is:
WHILE answer <> 'computer science' DO
SEND 'What is your favourite subject?' TO
DISPLAY
RECEIVE answer FROM (STRING) KEYBOARD
IF answer = 'computer science' THEN
SEND 'Good choice!' TO DISPLAY
ELSE SEND 'Really? ' TO DISPLAY
END IF
END WHILE
Pseudo-code is a simple way of describing a set of programming instructions in a manner that resembles a programming language.
Advantages and disadvantages of pseudo-code
Designing an algorithm in pseudo-code has advantages because:
-
it can be quickly and easily converted into an actual programming language as it is similar to a programming language
-
it is fairly easy to understand, even for non-programmers
-
it does not matter if there are errors in the syntax - it is usually still obvious what is intended
-
changes to the design can be incorporated quite easily
Pseudo-code also has its disadvantages:
-
It can be hard to see how a program flows. For example, where does following one path as opposed to another take the program?
-
It can be time consuming to produce.
Pseudo-code covers many areas of programming and there are different variants.
PART 5- ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 6- Sequence
Algorithms consist of instructions that are carried out (performed) one after another.
Sequencing is the specific order in which instructions are performed in an algorithm.
For example, a very simple algorithm for brushing teeth might consist of these steps:
-
put toothpaste on toothbrush
-
use toothbrush to clean teeth
-
rinse toothbrush
Each step is an instruction to be performed. Sequencing is the order in which the steps are carried out.
Why is sequencing important?
It is crucial that the steps in an algorithm are performed in the right order - otherwise the algorithm will not work correctly. Suppose the steps for the teeth-cleaning algorithm were in this sequence:
-
use toothbrush to clean teeth
-
put toothpaste on toothbrush
-
rinse toothbrush
A toothbrush would still be used to clean the teeth and toothpaste would still be put on the brush. But because steps 1 and 2 are in the wrong sequence the teeth wouldn’t get cleaned with the toothpaste, and the toothpaste would be wasted.
A human would realise they had forgotten to add toothpaste at the start of the process, but a computer would not know that anything was wrong.
PART 6- ACTIVITY >> WATCH THIS VIDEO (to 54 secs) >> DO THIS BBC QUIZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 7- Selection
A selection is used to make choices based on information. An algorithm can be made more intelligent by using IF, THEN and ELSE to repeat instructions or move to different parts of the program.
The algorithm about entering the room could be changed to account for different conditions. For instance, it could change to:
-
IF the door is locked, THEN unlock the door, ELSE do nothing (go to next instruction)
-
IF the door is closed, THEN open the door, ELSE do nothing
-
Enter the room
-
IF the room is dark, THEN switch on the light, ELSE do nothing
-
Close the door behind you
PART 7- ACTIVITY >> WATCH THIS VIDEO (54 secs to 2.40 min) >> DO THIS BBC QUIZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 8- Iteration
This repetition of a task is called iteration, and there are three main ways to approach it.
The WHILE loop
REPEAT (or DO) loop
FOR loop
There are two ways in which programs can iterate or ‘loop’:
-
count-controlled loops
-
condition-controlled loops
Each type of loop works in a slightly different way and produces different results.
Count-controlled loops
Sometimes it is necessary for steps to iterate a specific number of times.
Consider this simple algorithm for adding up five inputted numbers:
-
set the total to 0
-
repeat this section five times
-
input a number
-
add the number to the total
-
-
go back to step 2
-
say what the total is
This algorithm would allow five numbers to be inputted and would work out the total. Because it is known in advance how many times the algorithm needs to loop, a count-controlled loop is used.
Condition Controlled Loops
A condition-controlled loop is so called because iteration continues while, or until, a condition is met.
A condition controlled loop continues until a condition is met. At a race track, a car might be given the rules, loop until fuel run's out, then go to pitstop.
Consider this simple algorithm for entering a correct password:
enter password
unless password = “ilovecomputing”, go back to step 1
say ‘Password correct’
This algorithm would keep iterating until the password is entered correctly. A condition-controlled loop must be used because there is no way of knowing in advance how many times a password will need to be entered before it is correct.
PART 8- ACTIVITY >> WATCH THIS VIDEO (2.40 min to end) >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 9- Variables and Constants
A variable is a named memory location in random access memory (RAM) that holds a value. For more information on memory, see the memory study guide. The value held in a variable can - and usually does - change as the program is running.
A variable's name is known as an identifier. The identifer given to a variable usually follows certain rules:
-
It can contain letters and numbers but must start with a letter.
-
It must contain at least one letter (at the start of the name).
-
It must not contain special characters such as !@£$%&* or punctuation characters. However, an underscore can be used. Spaces are not allowed.
-
The name should be meaningful - it should represent the value it is holding.
Acceptable variable identifiersUnacceptable variable identifiers
highScorehigh score (cannot contain a space)
high_score123score (must start with a letter)
highScore123$core (must start with a letter)
Variables make it easy for a program to store values in memory locations when it is running. The computer keeps track of which memory location the variable refers to. The programmer simply has to remember the name of the identifier the variable was given.
Declaration and assignment
Some programming languages require a variable to be declared before a value is assigned to it. How this is done varies by programming language. A variable does not need to be declared in pseudo-code before using it. However, when using real programming language it is good practice to always declare a variable before it is used. This ensures that a memory location is ready to hold a value.
For example, in Visual Basic.NET the instruction:
Dim score as integer
would declare a variable called score which will hold integer values.
Giving a variable a value is known as assignment. A variable must be assigned a value before it can be used. For example:
score = 0
would assign the value 0 to the variable score.
Some programming languages enable variables to be declared and assigned a value in the same line of code. For example:
Dim score as integer = 0
Constants
A constant enables a value to be assigned a name. Unlike a variable, the value assigned to a constant cannot be changed while the program is running.
Constants are useful because they are declared and assigned once, but can be referred to over and over again throughout the program. They are used for values that are unlikely to change, for example:
-
Pi, eg const PI = 3.142
-
VAT, eg const VAT = 20
Constants follow the same naming conventions as variables, except that they are usually in uppercase. This helps to make the programmer aware that they are accessing a constant rather than a variable.
Global and local variables
The area of a program where a variable is accessible is referred to as its scope. A global variable can be accessed and changed throughout the whole program. It is declared outside of a subprogram.
Local variables are usually confined to a subprogram. A variable with local scope will only be available within the subprogram and it is declared within the subprogram. Therefore, the programmer is able to use the same variable names again and again for different purposes. This makes debugging easier as programmers know what section of code has access to the variables.
When using global variables, programmers must be careful not to use a local variable with the same name. Depending on the programming language, this could result in an error or the programmer updating the wrong version (local or global) of the variable. In general, global variables should be avoided as much as possible. This is because the value of the variable could be changed anywhere within the program and this makes debugging very difficult.
Python works slightly differently and a global variable has to be declared as such within a subprogram before it can be used.
For example, in Python you would use ‘global ID = 75’ within a function to access the global variable ‘ID’.
PART 9- ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 10- Strings, Records, Arrays
Strings
A string is a variable that holds a sequence of one or more alphanumeric characters. It is usually possible to manipulate a string to provide information or to alter the contents of a string.
The following examples use strings:
wordOne = "Computer" wordTwo = "Science"
Length
The length of a string can usually be determined using the len statement. This gives the length as an integer.
len(wordOne) - would give the answer eight, as there are eight characters in the word "Computer"
Character position
It is possible to determine which character features at a position within a string:
wordOne[2] - would give the answer "m", as "m" is the third character in the word “Computer” (remember computers start counting at zero)
wordOne[0:2] - would give "Com", the first three characters in the string
wordOne[3:3] - would give "put", the three characters starting from position three
Upper and lowercase
It is possible to change all letters in a string to either lowercase or uppercase. This can be very useful, for example when checking possible inputs:
topic = "Computer Science"
topic = topic.lower - would give a value for the topic of "computer science"
topic = topic.upper - would give a value for the topic of "COMPUTER SCIENCE"
Concatenation
To concatenate strings means to join them to form another string. For example:
sentence = wordOne + " " + wordTwo - would give "Computer Science"
Alternatively, a string can be lengthened by adding more characters. For example:
wordOne = wordOne + " Science" - would result in the value of wordOne becoming "Computer Science"
PART 10- STRINGS ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
Records
A record is a data structure that groups together related items of data. These are slightly more complex than arrays as you can store more than one type of data together. For example, with a game, it could be useful to set up a data structure which collects a player's login and their score in one structure.
Creating records will vary in different languages. Python uses a data structure called 'dictionaries' that has some features of the record structure.
Example
In this example we have set up a structure which defines a player's name and then captures their login and score as one record. We have two players, Katie and Patrick. We will use the record to capture the player names Katie and Patrick with their logins and scores. Katie's login is kat10 and her score is 124. Patrick's login is pat00 and his score is 99.
This line defines a dictionary data structure with two records of a player's login name and score. The precise use of the syntax such as ‘:’ and ‘{‘ brackets makes this possible.
>>> players = { 'Katie' : { 'login': 'kat10', 'score' : 124 }, ‘Patrick' : { 'login': 'pat00', 'score' : 99 } }
The next line adds a new record to the dictionary:
>>> players['Tom'] = { 'login': 'tom13', 'score' : 121 }
The following code should return all three records in the dictionary:
>>> players
And this line of code would remove Katie's score:
>> del players['Katie']
PART 10- RECORDS ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
Arrays & Lists
An array is one method of storing data in an organised structure.
Imagine you were making a game and you wanted to store player names and their scores. You would need to write some code to tell the program to store this information.
When arrays are created, the program needs to know their size. It needs to know how many player names and scores you want to collect. When an array or any variable is created in a program this is called declaring. For example, if you created two arrays which could store five pieces of data each for player scores and player names, they would be declared with the following:
-
gameScores[5]
-
playerNames[5]
An array stores a set of data elements in a certain order. A data element is any single unit of data within a program. You can tell the program exactly what the names and scores are by writing the following:
-
gameScores = (124, 99, 121, 105, 132)
-
playerName = ("Katie","Patrick","Tom","Rosie","Michael")
Arrays do not store mixed data types. The first array is a collection of numbers showing the five scores. Each data element is an integer. The second array is a collection of names. Each element is a character string.
Each element in an array relates to a specific place in memory. You could imagine this to be like shelves in a cupboard. The array is the cupboard with shelves inside it, and each shelf is numbered and used to hold a specific item.
A one-dimensional array can be seen as data elements organised in a row. A two-dimensional array is similar to a one-dimensional array, but it can be visualised as a grid (or table) with rows and columns.
For example, a nine-by-nine grid could be referenced with numbers for each row and letters for each column. A nine-by-nine, two-dimensional array could be declared with a statement such as:
game [9][9]
Many games use two dimensional arrays to plot the visual environment of a game. Positions in a two dimensional array are referenced like a map using horizontal and vertical reference numbers. They are sometimes called matrices.
PART 10- ARRAYS ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
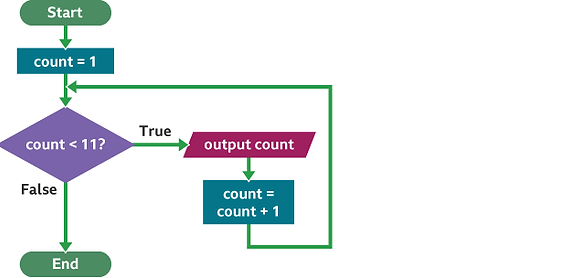
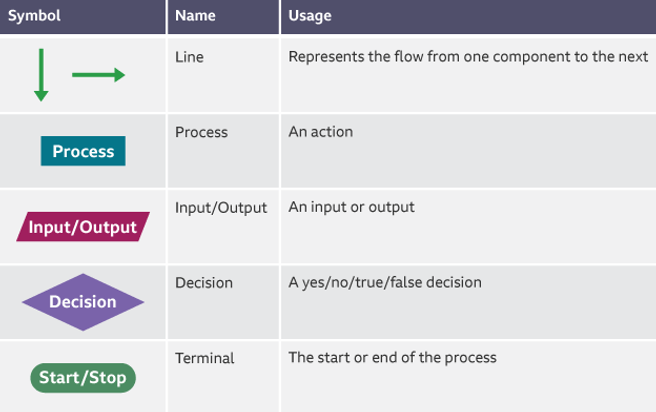
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 11- Operators (Boolean, Logic, Math)
An operator is a character, or characters, that determine what action is to be performed or considered.
There are three types of operator that programmers use:
-
mathematical operators
-
logical operators
-
Boolean operators
These operators are common to most high level programming languages.
Mathematical operators
Computers are designed to carry out calculations. Mathematical operators allow arithmetic to be performed on values.
Mathematical operationOperatorExample
Addition+x = x + 5
Subtraction-x = x - 5
Multiplication*x = x * 5
Division/x = x / 5
Integer divisionDIVx = x DIV 5
RemainderMODx = x MOD 5
PART 11 MATH. ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
Logical or Comparison operators
Logical operators allow for assignment and for comparisons to be made. They are used in condition testing.
Logical operationOperatorExample
Assignment=x = 5
Equivalence= or ==if x = 5 or if x == 5
Less than<if x < 5
Less than or equal to<=if x <= 5
Greater than>if x > 5
Greater than or equal to>=if x >= 5
Does not equal<> or !=If x <> 5 or if x != 5
PART 11 LOGIC ACTIVITY >> WATCH THIS VIDEO (from 3.22) >> DO THIS QUIZIZZ >> DO THESE ACTIVITIES (DO TASKS x,x)
Boolean operators
Boolean operators are used to combine logical operators to give more complex decisions.
Boolean operationOperatorExample
AndANDif x > 0 AND x < 10
OrORif topic == "Computing" OR topic == "Computer Science"
NotNOTwhile NOT x
PART 11 BOOLEAN ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 12- Testing, Trace Tables and Errors
Testing
Testing is one of the most important processes when creating new software. Without testing a new system thoroughly it can fail, resulting in serious consequences for the developers and end users.
It can be difficult to test every possible outcome and even the most rigorous of testing may not catch every error in the program. There are many examples of situations where new computer systems or software upgrades have caused major problems because they have not been tested properly.
Testers actively try to break the system, pushing it to extremes to test its strength and resilience.
TASK 12 TESTING ACTIVITY >> WATCH THIS VIDEO >> DO THIS BBC QUIZ >> ACTIVITIES HERE
Trace Tables
When testing more complex examples of software, it is sometimes necessary to test a number of conditions and sub-conditions at the same time. A trace table, also called a trace matrix, can be used to record the outcomes of the test. The trace table for a very simple example, such as x=y+2, would look like this:
y x
13 15
25 27
1200 1202
A trace table might appear to look very similar to a test plan, but without the other headings like 'expected outcome' and 'actual outcome'.
TASK 12 TRACE TABLES ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
Syntax, execution and logic errors
There are three types of error that you need to understand.
Syntax error
This is an error in the spelling or grammar used when coding. Missing a letter, character or forgetting to include inverted commas/speech marks are common examples of syntax errors. A syntax error will be identified by an interpreter as it will be unable to convert the source code into machine code.
Execution error
Sometimes called a runtime error, execution errors only become evident during run time. An execution error occurs when a program is asked to do something that it cannot, resulting in a ‘crash’. The widely used example of a run time error is asking a program to divide by 0.
The code contains no syntax or logic errors but when it runs it can't perform the task that it has been programmed to carry out. Another example is where an attempt is made to access an item in an array which does not exist eg item 11 in an array with only ten elements.
File handling can also result in execution errors, most often when an attempt is made to write to a file that does not exist.
Logic error
An error in the logic of the code such as using ˂ instead of ˃ or AND instead of OR. The program will execute the code but will produce unexpected results. Logic errors are usually resolved by carrying out a dry run, using a trace table or setting breakpoints to help identify the section of code that contains the logic error.
TASK 12 ERROR ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 13- Boolean Algebra and Truth Tables
Boolean
The Boolean data type has only two values: true or false. These values are used to control the flow of the execution of programs. Boolean values are found by comparing other data values. The results of these comparisons may be combined in Boolean expressions.
Logic gates
Many electronic circuits have to make decisions. They look at two or more inputs and use these to determine the outputs from the circuit. The process of doing this uses electronic logic, which is based on digital switches called gates.
Logic gates allow an electronic system to make a decision based on a number of its inputs. They are digital electronic devices. Each input and output of the gates must be one of two states:
-
true or 1 or 'on'
-
false or 0 or 'off'
A single digital signal can be either on or off - for example, a light with one switch can be on or off. However, if there is more than one signal, there are more than two possible states. For example, if two signals are present there are four possible combinations: on/on, on/off, off/on and off/off.
In a logic gate, each combination can be made to produce a different outcome. Binary numbers reflect the two states - on and off, 1 and 0, true and false - within CPU transistors. Logic gate calculations can also be represented as truth tables.
PART 13- LOGIC GATES ACTIVITY >> WATCH THIS VIDEO >> PLAY WITH LOGIC GATES >> DO THIS QUIZIZZ >> ACTIVITIES HERE
Truth Tables
Truth tables are a way of showing all the possible outputs for inputs in a logical expression. Logic gates, used in electronics and therefore computer circuits, are based on truth tables. More complex expressions can combine several inputs and outputs. In the following tables, 1 means TRUE and 0 means FALSE.
PART 13- TRUTH TABLE ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 14- Algorithms In Action- Searches and Sorts
Since computers were created, users have devised programs, many of which, in part, needed to do the same thing. As a result, standard algorithms have evolved and been adopted in many programs.
Two types of algorithm that are often used are searches and sorts. Searches enable a data set to be examined and a specific item to be found. Sorts enable a data set to be sorted into order.
Standard searching algorithms include:
-
linear search
-
binary search
Standard sorting algorithms include:
-
bubble sort
-
merge sort
LINEAR SEARCH
A linear search is the simplest method of searching a data set.
Starting at the beginning of the data set, each item of data is examined until a match is made. Once the item is found, the search ends. If there is no match, the algorithm must deal with this.
A written description algorithm for a linear search might be:
-
Find out the length of the data set.
-
Set counter to 0.
-
Examine value held in the list at the counter position.
-
Check to see if the value at that position matches the value searched for.
-
If it matches, the value is found. Send a message and end the search.
-
If not, increment the counter by 1 and go back to step 3 until there are no more items to search.
-
If all the items have been checked and no match is found, send a message.
BINARY SEARCH
A binary search is an efficient method of searching an ordered list. It will not work on a list that has not been sorted first.
A written description of a binary search algorithm is:
-
Start by setting the counter to the middle position in the list.
-
If the value held there is a match, the search ends and a message is sent.
-
If the value at the midpoint is less than the value to be found, the list is divided in half, the lower half of the list is ignored and the search keeps to the upper half of the list.
-
Otherwise, if the value at the midpoint is greater than the value to be found, the upper half of the list is ignored and the search keeps to the lower half of the list.
-
The search moves to the midpoint of the remaining items. Steps two to four continue until a match is made or there are no more items to be found and a message is sent.
PART 14- LINEAR SEARCH ACTIVITY >> WATCH THIS VIDEO >> ACTIVITIES HERE
PART 14- BINARY SEARCH ACTIVITY >> WATCH THIS VIDEO >> ACTIVITIES HERE
SEARCH QUIZ- QUIZIZZ
BUBBLE SORT
A bubble sort is the simplest of the sorting algorithms. However, it is an inefficient sort for anything but a small list because of the number of comparisons required.
A written description algorithm of a bubble sort is:
-
Start at the beginning of the list.
-
Compare the first value in the list with the next one up. If the first value is bigger, swap the positions of the two values.
-
Move to the second value in the list. Again, compare this value with the next and swap if the value is bigger.
-
Keep going until the there are no more items to compare. Note - the last item checked in the list is now sorted, so ignore this next time.
-
Go back to the start of the list.
Each run through the list, from start to finish, is known as a pass. The bubble sort continues until a pass is made where no values have been swapped. At this point, the list is sorted.
MERGE SORT
A merge sort is a more complex sort, but also a highly efficient one.
A merge sort uses a technique called divide and conquer. The list is repeatedly divided into two until all the elements are separated individually. Pairs of elements are then compared, placed into order and combined. The process is then repeated until the list is recompiled as a whole.
PART 14- BUBBLE SORT ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
PART 14- MERGE SORT ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE
------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------
PART 15- Sub Programs- Procedures and Functions
Subprograms are small programs that are written within a larger, main program. The purpose of a subprogram is to perform a specific task. This task may need to be done more than once at various points in the main program.
There are two types of subprogram:
-
procedures
-
functions
Benefits of using subprograms
-
Subprograms are usually small in size, meaning they are much easier to write, test and debug. They are also easy for someone else to understand.
-
Subprograms can be saved separately as modules and used again in other programs. This saves time because the programmer can use code that has already been written, tested and debugged.
-
A subprogram may be used repeatedly at various points in the main program. However, the code only has to be written once, resulting in shorter programs.
Procedures
A procedure is a subprogram that performs a specific task. When the task is complete, the subprogram ends and the main program continues from where it left off. For example, a procedure may be written to reset all the values of an array to zero, or to clear a screen.
A procedure is created using the following syntax:
procedure identifier (value to be passed) procedure code endprocedure
This procedure would clear the screen by printing x blank lines:
procedure clear_screen(x) for i = 1 to x: print(" ") endprocedure
A procedure is run by calling it. To call it, a programmer uses the procedure name and includes any values that the procedure needs, for example:
clear_screen(5)
This would print five blank lines on the screen.
Functions
A function works in the same way as a procedure, except that it manipulates data and returns a result back to the main program.
For example, a function might be written to turn Fahrenheit into Celsius:
function f_to_c(temperature_in_f) temperature_in_c= (temperature_in_f –32) * 5/9 return temperature_in_c endfunction
A function is run by calling it. To call it, a programmer uses the function's identifier, the value to be passed into the function, and a variable for the function to return a value into, for example:
celsius = f_to_c(32)
This would result in the value of Celsius being zero.
Procedures perform a specific task. Functions manipulate data and return a value to the main program.
Built-in functions
Many languages include built-in, ready-made functions:
-
int - converts strings or floats into integers
-
str - converts a number into a string
-
asc - finds the ASCII number of a character
Additionally, some languages allow functions to be added in from external files called libraries. Libraries contain pre-written, tested functions that extend the functionality of a language.
PART 15 ACTIVITY >> WATCH THIS VIDEO >> DO THIS QUIZIZZ >> ACTIVITIES HERE